JSON
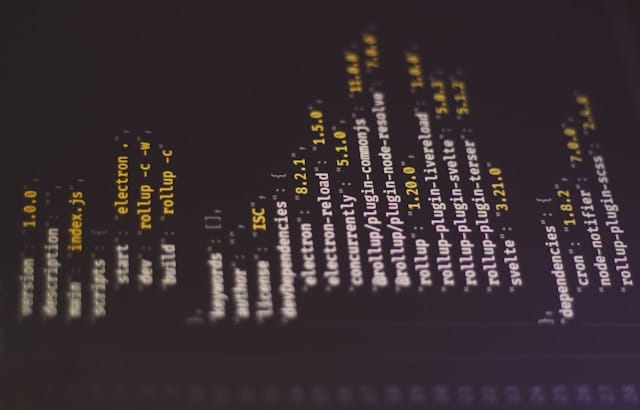
Why do you have to have a basic understanding of JSON?
Many of the APIs exchange data in JSON format. If you are consuming APIs or developing APIs, it is good to understand this simple format.
JSON(JavaScript Object Notation) is a text format adhering to a convention. In layman's terms, you can open a text editor like Notepad and write text that adheres to the JSON convention. It is typically used for APIs to return data or accept data.
Three basic thing to know to get you going with JSON.
- A JSON object is comma-separated collection of key-value pairs within a single pair of curly braces.
- A JSON array is a comma separated collection of JSON objects withing a single pair of square brackets.
- You can nest JSON objects. That is, you can provide another JSON objects as a value to a key.
Example below will demystify these points.
{"family_id":"1","biological_father":"Jarli Arika", "biological_mother":"Ngarra Yindi","child_1":"Djin-Djin Koa","child_2":"Kali Nyari"}
A prettier way to write it:
{
"family_id": "1",
"biological_father": "Jarli Arika",
"biological_mother": "Ngarra Yindi",
"child_1": "Djin-Djin Koa",
"child_2": "Kali Nyari"
}
The whitespaces and newlines are optional. If your value has a double quote in it, you can escape it like this:
{
"first_name": "Jarli \"s",
"last_name": "Arika"
}
Single quote is not a problem.
{
"first_name": "Jarli's",
"last_name": "Arika"
}
The advantage with JSON is human readability and understandability. Just looking at the data we know that the API is returning family data.
A JSON array allow you to provide a collection of JSON objects.
[
{
"family_id": "1",
"biological_father": "Jarli Arika",
"biological_mother": "Ngarra Yindi",
"child_1": "Djin-Djin Koa",
"child_2": "Kali Nyari"
},
{
"family_id": "2",
"biological_father": "Nullah Oodgeroo",
"biological_mother": "Yani Maiara",
"child_1": "Makani Marrin",
"child_2": "Jumbun Djaran"
}
]
Note: Although JSON array is a completely valid way of sending data in an API, it is better to wrap it as a JSON object.
{
"data": [
{
"family_id": "1",
"biological_father": "Jarli Arika",
"biological_mother": "Ngarra Yindi",
"child_1": "Djin-Djin Koa",
"child_2": "Kali Nyari"
},
{
"family_id": "2",
"biological_father": "Nullah Oodgeroo",
"biological_mother": "Yani Maiara",
"child_1": "Makani Marrin",
"child_2": "Jumbun Djaran"
}
]
}
Now let us understand how nesting of data in JSON looks like.
To accommodate families with more than two children, we might have to add additional keys like child3, child4 and so on. Instead we can provide a collection of children in a children key.
{
"data": [
{
"family_id": "1",
"biological_father": "Jarli Arika",
"biological_mother": "Ngarra Yindi",
"children": [
{
"name": "Djin-Djin Koa"
},
{
"name": "Kali Nyari"
}
]
},
{
"family_id": "2",
"biological_father": "Nullah Oodgeroo",
"biological_mother": "Yani Maiara",
"children": [
{
"name": "Makani Marrin"
},
{
"name": "Jumbun Djaran"
}
]
}
]
}
We can make it a little bit facier
{
"data": [
{
"family_id": "1",
"biological_father": {
"first_name": "Jarli",
"last_name": "Arika",
"dob": "1/1/1999",
"good_parent": true
},
"biological_mother": {
"first_name": "Ngarra",
"last_name": "Yindi",
"dob": "1/1/1999",
"good_parent": null
},
"children": [
{
"name": "Djin-Djin Koa"
},
{
"name": "Kali Nyari"
}
]
},
{
"family_id": "2",
"biological_father": {
"first_name": "Nullah",
"last_name": "Oodgeroo",
"dob": "1/1/1989"
},
"biological_mother": {
"first_name": "Yani",
"last_name": "Maiara",
"dob": "1/1/1987"
},
"children": [
{
"order": 1,
"name": "Makani Marrin"
},
{
"order": 2,
"name": "Jumbun Djaran"
}
]
},
{
"family_id": "3",
"biological_father": {
"first_name": "Chebona",
"last_name": "Bula",
"dob": "1/1/2001"
},
"biological_mother": {
"first_name": "Halona",
"last_name": "Scenanki",
"dob": "1/1/2000"
},
"children": []
}
]
}
Few things to note:
- Arrays can be empty. Observe the value for children for the family id 3.
- You do not have to have all keys consistently in all objects. For eg., the parent with family id of 2 has good_parent key. Other parents do not have this key.
- keys names are arbitrary. You as an api developer determine what the key name should be. You determine what to send across.
- The values can be null, boolean(true,false), string, number or another JSON Object or JSON array.
- For family_id, the integer numbers are represented as strings. If they are meant to be numbers, it is better to remove the doublequotes and provide the numeric value. Compare it with the integer values provided for the order key of the children.