Python Functions - Define and return values
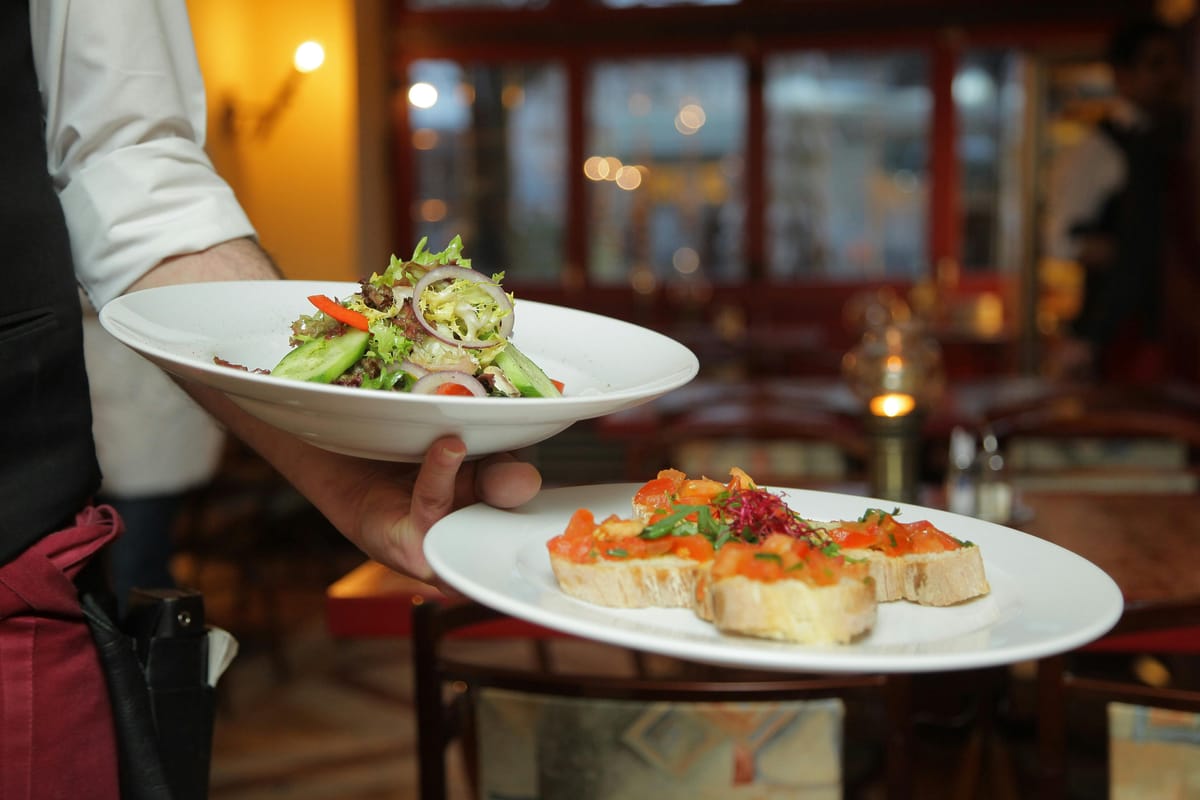
In this post I am going to explain how to create a function and how to invoke it.
You can use the def keyword to create/ define a function as follows. I have created two functions called func1 and func2 below. If I do not want to fully flesh out the logic for my function, I can use the pass keyword or ellipsis(...). The code within the function definition block is not executed until the function is invoked. Below the functions are merely being defined.
function_lesson.py
def func1():
#list the steps or logic here
pass
def func2():
#list the steps or logic here
pass
You invoke then by calling them as follows:
def func1():
#list the steps or logic here
pass
def func2():
#list the steps or logic here
pass
#I am no going to invoke the functions
func1()
func2()
You might want the Functions to take parameter values as input to use in the logic. The actual values are passed when you actually invoke the function.
def func1():
#list the steps or logic here
pass
def func2():
#list the steps or logic here
pass
#I am no going to invoke the functions
func1()
func2()
#defining a function that take a parameter
def func3(customername):
print(f'I am {customername}.')
#defining a function that takes multiple parameter
def func4(first_name, last_name):
print(f'I am {last_name}.{first_name} {last_name}.')
#You can invoke a function that take a parameter
#by passing the actual value for the parameter
func3('Bond')
func4('James','Bond')
The output is as follows:

A function can return multiple value as a tuple. Let us explore a scenario where you might use it. Let us say you write a program that take your first and last name as command-line parameters and prints Hello Lastname, Firstname. Take the following example
import sys
def print_command_line_arguments():
# Print the script name
print(f"Script name: {sys.argv[0]}")
# Print other command-line arguments
for i, arg in enumerate(sys.argv[1:], start=1):
print(f"Argument {i}: {arg}")
def read_command_line_arguments_return_tuple():
#check whether the user has provided the first name and last name
if len(sys.argv) < 3:
print("Please provide the first name and last name as command-line arguments")
sys.exit(1)
first_name = sys.argv[1]
last_name = sys.argv[2]
#return them as a tuple
return first_name, last_name
def read_command_line_arguments_return_dictionary():
#check whether the user has provided the first name and last name
if len(sys.argv) < 3:
print("Please provide the first name and last name as command-line arguments")
sys.exit(1)
first_name = sys.argv[1]
last_name = sys.argv[2]
#return them as a dictionary
return {"first_name":first_name, "last_name": last_name}
def read_command_line_arguments_return_list():
#check whether the user has provided the first name and last name
if len(sys.argv) < 3:
print("Please provide the first name and last name as command-line arguments")
sys.exit(1)
first_name = sys.argv[1]
last_name = sys.argv[2]
#return them as a tuple
return [first_name, last_name]
def main():
print_command_line_arguments()
first_name, last_name= read_command_line_arguments_return_tuple()
print(f"Hello {last_name}, {first_name}!")
name_dict = read_command_line_arguments_return_dictionary()
print(f"Bonjour {name_dict['last_name']}, {name_dict['first_name']}!")
name_list = read_command_line_arguments_return_list()
print(f"Hallo {name_list[1]}, {name_list[0]}!")
if __name__ == "__main__":
main()
The output is as follows:
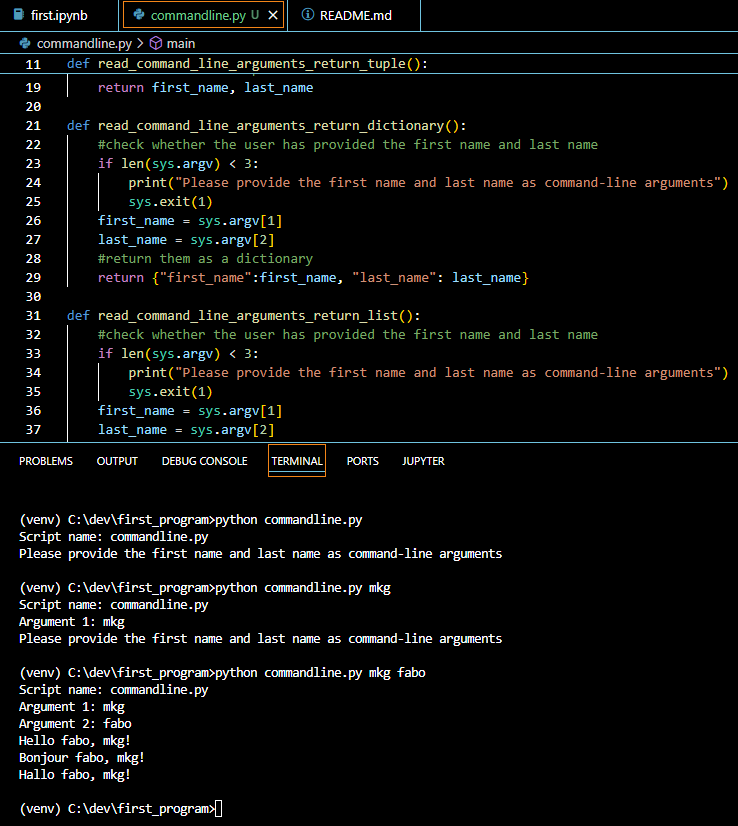