API Tutorial
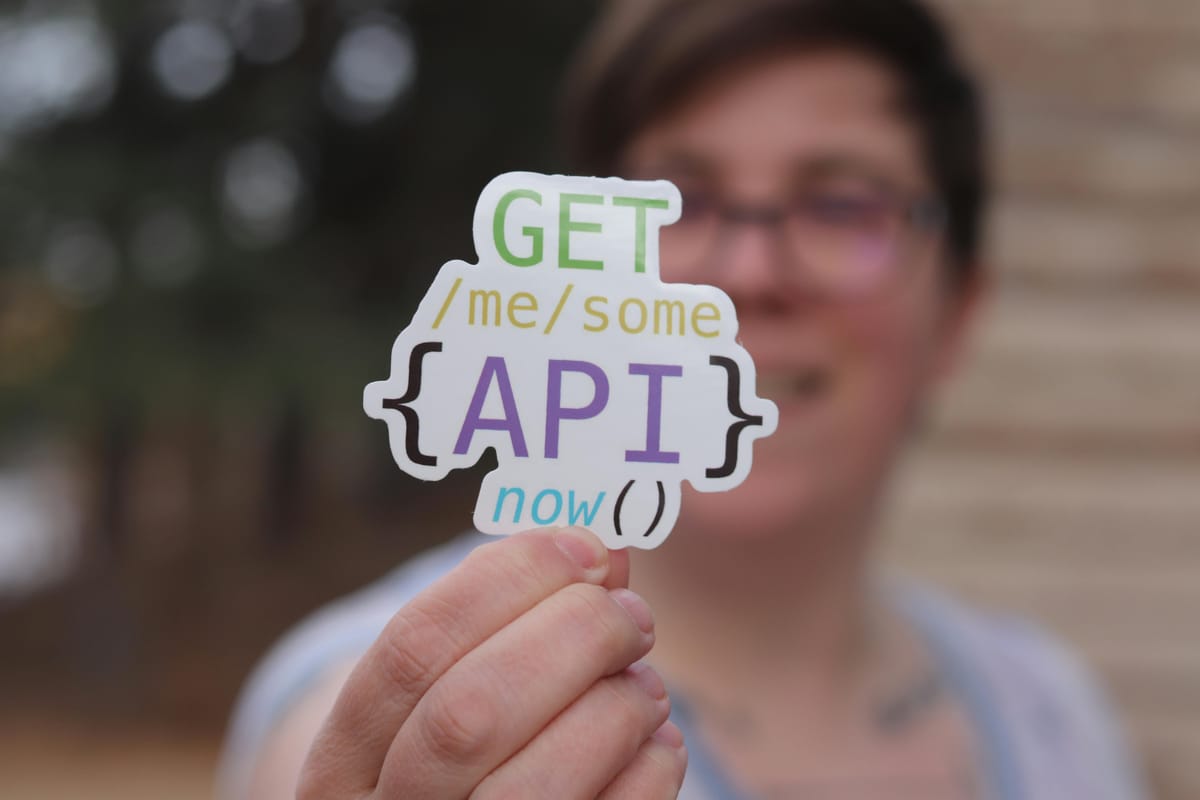
- Why do we need APIs?
APIs (Application Programming Interfaces) are necessary for several reasons:
- Security: APIs act as a barrier between your database and the outside world, controlling access and protecting sensitive data.
- Scalability: APIs can handle multiple requests efficiently and can be easily scaled.
- Flexibility: APIs allow different systems and programming languages to communicate.
- Standardization: APIs provide a consistent way to interact with data across different platforms.
- Why can't you connect directly to databases?
While it's technically possible to connect directly to databases, it's generally not recommended for several reasons:
- Security risks: Direct database access could expose sensitive data or allow unauthorized modifications.
- Performance issues: Databases might not handle numerous concurrent connections well.
- Lack of business logic: APIs can implement complex business rules and data validations.
- Versioning and maintenance become more difficult.
- How HTTP methods correspond to database operations:
- GET ≈ SELECT: Retrieve data
- POST ≈ INSERT: Create new data
- PUT/PATCH ≈ UPDATE: Modify existing data
- DELETE ≈ DELETE: Remove data
Remember, these are approximate correspondences. APIs often involve more complex operations that may combine multiple.
Key Concepts in RESTful APIs:
- Resources: In REST, everything is considered a resource. This could be a user, a book, an order, etc. Each resource is identified by a unique URI (Uniform Resource Identifier).
- HTTP Methods: REST uses standard HTTP methods to perform operations on resources:
- GET: Retrieve a resource
- POST: Create a new resource
- PUT: Update an existing resource
- DELETE: Remove a resource
- PATCH: Partially modify a resource
- Representations: Resources can have multiple representations. For example, a user resource might be represented in JSON, XML, or HTML. To keep it simple we will assume all representations will be in JSON.
GET vs. POST
GET and POST are two of the most commonly used HTTP methods in RESTful APIs. Let's break down each of them:
GET:
- Purpose: GET is used to retrieve data from a specified resource.
- Characteristics:
- Idempotent: Multiple identical requests should have the same effect as a single request.
- Safe: Should not change the state of the server.
- Can be cached
- Can be bookmarked
- Remains in browser history
- Has length restrictions
- Should only retrieve data, not modify it
- Data Transmission:
- Data is sent as part of the URL
- Visible in the URL (not secure for sensitive data)
- Limited amount of data can be sent (max URL length)
- Example: GET /api/books?genre=fiction&sort=titleThis might retrieve a list of fiction books, sorted by title.
- Typical Use Cases:
- Fetching a list of resources (e.g., list of books)
- Retrieving a specific resource (e.g., details of a particular book)
- Performing searches
POST:
- Purpose: POST is used to submit data to be processed to a specified resource.
- Characteristics:
- Not idempotent: Multiple identical requests may result in multiple resource creations
- Not safe: Changes the state of the server
- Cannot be cached
- Cannot be bookmarked
- Does not remain in browser history
- No data length restrictions
- Data Transmission:
- Data is sent in the request body
- Not visible in the URL (more secure for sensitive data)
- Can send large amounts of data
- Example: POST /api/books { "title": "1984", "author": "George Orwell", "genre": "Dystopian Fiction" }This might create a new book in the system.
- Typical Use Cases:
- Creating a new resource (e.g., adding a new book)
- Submitting form data
- Uploading a file
- Adding a child resource (e.g., adding a comment to a blog post)
Key Differences:
- Data in URL vs Body: GET sends data as part of the URL, POST sends it in the request body.
- Data Visibility: GET data is visible in the URL, POST data is not.
- Data Amount: GET has limitations on data amount, POST does not.
- Caching: GET requests can be cached, POST requests are not typically cached.
- Idempotency: GET is idempotent, POST is not.
- Security: POST is more secure for sensitive data as it's not visible in the URL.
- Purpose: GET is for retrieving data, POST is for submitting data to be processed.
In your API design, you would typically use GET for operations that don't change server state and POST for operations that do. Understanding the differences and appropriate uses of these methods is crucial for designing RESTful APIs that adhere to best practices and conventions.
RECAP
Understanding APIs and REST
- What is an API?
API stands for Application Programming Interface. Think of it as a waiter in a restaurant:
- You (the client) don't go directly into the kitchen (the database).
- Instead, you tell the waiter (the API) what you want.
- The waiter goes to the kitchen, gets what you need, and brings it back to you.
APIs allow different software systems to communicate with each other safely and efficiently.
- Why do we need APIs?
Imagine if everyone could walk into the restaurant kitchen whenever they wanted. It would be chaotic and unsafe! Similarly, APIs are crucial because they:
- Provide security by controlling access to your data
- Allow for efficient handling of multiple requests
- Enable different systems (like a website and a mobile app) to communicate with the same backend
- Standardize how data is requested and received
- REST: A Way to Design APIs
REST (Representational State Transfer) is a popular way to design APIs. It's like a set of rules for how the waiter should behave in our restaurant analogy.
Key REST Principles:
- Client-Server: The client (e.g., a mobile app) and server (where the API lives) are separate.
- Stateless: Each request contains all the information needed. The waiter doesn't remember your previous orders.
- Cacheable: Responses say whether they can be cached (saved for quick access later) or not.
- Uniform Interface: There's a standard way to ask for things, no matter what you're asking for.
- HTTP Methods: The Language of REST
In REST, we use HTTP methods to perform different actions. Think of these as the different ways you can interact with the waiter:
- GET: Ask for information (e.g., "What's on the menu?")
- POST: Send new information (e.g., "I'd like to place an order.")
- PUT/PATCH: Update existing information (e.g., "Actually, can I change my order?")
- DELETE: Remove information (e.g., "I'd like to cancel my order.")
- Resources and Endpoints
In REST, everything is a resource (like dishes on a menu). Each resource has a unique address called an endpoint (like a table number). For example:
- /api/books might list all books
- /api/books/123 might give details about the book with ID 123
- Request and Response
When you interact with an API:
- You send a request (like asking the waiter for something)
- You get back a response (like the waiter bringing what you asked for)
Let's look at two common types of requests:
GET Request:
- Used to retrieve information
- Data is sent as part of the URL
- Example: GET /api/books?genre=fiction
- This is like asking the waiter, "Can I see all the fiction books you have?"
POST Request:
- Used to send new information
- Data is sent in the request body, not in the URL
- Example: POST /api/books { "title": "New Book", "author": "Jane Doe" }
- This is like telling the waiter, "I'd like to add this new book to your collection."
- Status Codes
APIs use status codes to tell you what happened with your request:
- 200 OK: Everything worked fine
- 201 Created: Your POST request was successful
- 400 Bad Request: You made a mistake in your request
- 404 Not Found: The resource you asked for doesn't exist
- 500 Internal Server Error: Something went wrong on the server's end
- Authentication and Authorization
Many APIs require you to prove who you are (authentication) and check what you're allowed to do (authorization). It's like having a membership card at an exclusive restaurant.
- Advanced Concepts
As you get more comfortable with APIs, you'll encounter more advanced concepts:
- Pagination: Getting results in smaller chunks (like pages in a book)
- Filtering and Sorting: Asking for specific items or in a particular order
- Relationships: How different resources connect (like authors and their books)
- Versioning: How APIs handle changes over time
- Building an API
When you're ready to build your own API, start simple:
- Define your resources (e.g., books, authors)
- Create endpoints for each resource
- Implement the basic HTTP methods (GET, POST, PUT, DELETE)
- Add error handling and appropriate status codes
- Test your API thoroughly
As you progress, you can add more complex features like search functionality, authentication, and advanced querying options.
Project
This will serve as a practical application of the concepts we've discussed.
- Library Management System API
Now that we've covered the basics of APIs and REST, let's put this knowledge into practice by creating a Library Management System API. This project will help you understand how different components of an API work together.
Assignment Objective: Create a RESTful API for a library system that manages books, users, authors, and booklists, with a search functionality.
Entities:
- Books
- Users
- Authors
- Booklists (curated lists of books)
Main API Endpoints to Implement:
- Books:
- GET /api/books - List all books
- GET /api/books/{id} - Get a specific book
- POST /api/books - Add a new book
- PUT /api/books/{id} - Update a book
- DELETE /api/books/{id} - Delete a book
- Users:
- GET /api/users - List all users
- GET /api/users/{id} - Get a specific user
- POST /api/users - Add a new user
- PUT /api/users/{id} - Update a user
- DELETE /api/users/{id} - Delete a user
- Authors:
- GET /api/authors - List all authors
- GET /api/authors/{id} - Get a specific author
- POST /api/authors - Add a new author
- PUT /api/authors/{id} - Update an author
- DELETE /api/authors/{id} - Delete an author
- Booklists:
- GET /api/booklists - List all booklists
- GET /api/booklists/{id} - Get a specific booklist
- POST /api/booklists - Create a new booklist
- PUT /api/booklists/{id} - Update a booklist
- DELETE /api/booklists/{id} - Delete a booklist
- POST /api/booklists/{id}/books - Add a book to a booklist
- DELETE /api/booklists/{id}/books/{bookId} - Remove a book from a booklist
- Search Functionality:
- GET /api/search/books?author={authorName} - Search books by author name
Additional Requirements:
- Implement relationships between entities:
- Books should be associated with one or more authors
- Users can create and manage booklists
- Booklists contain multiple books
- Add pagination for list endpoints (e.g., GET /api/books?page=1&limit=20)
- Implement filtering and sorting (e.g., GET /api/books?genre=fiction&sort=title)
- Use appropriate HTTP status codes and implement error handling
- Add basic authentication:
- Users must be authenticated to create booklists
- Only administrators can add/update/delete books and authors
- Implement search by author functionality with partial matches and case-insensitive searches
- Create an endpoint to get all books by a specific author (e.g., GET /api/authors/{id}/books)
- Implement a feature to get recommended books based on a user's booklists
Advanced Challenges (Optional):
- Add a rating system for books and create an endpoint to get top-rated books
- Implement a book borrowing system with due dates and overdue notifications
- Create a more advanced search that looks through books, authors, and booklists
- Add an endpoint to generate reports (e.g., most popular books and authors)
- Implement API versioning (e.g., /api/v1/books, /api/v2/books)
This assignment covers all CRUD operations, involves multiple related resources, and includes more advanced features like search functionality and recommendations. It will give you hands-on experience with RESTful API design and implementation, as well as practice in handling relationships between different entities in your system.
Remember to approach this project step-by-step:
- Start by setting up your development environment
- Create your basic entity models
- Implement the main CRUD operations for each entity
- Add the relationships between entities
- Implement the search functionality
- Add authentication and authorization
- Finally, work on the advanced features
Step 1: Set up the environment
- Install FastAPI and dependencies:
`pip install fastapi uvicorn sqlalchemy pydantic`
- Create a new directory for your project and navigate into it:
`mkdir library_api
cd library_api`
Step 2: Create the basic structure
Create the following files:
- main.py
- models.py
- schemas.py
- database.py
Explanation of the files:
- database.py
Imagine this file as the blueprint for a filing cabinet. It doesn't contain any actual information yet, but it sets up how we're going to store and organize our data. It's like deciding:
- What brand of filing cabinet we'll use (in this case, a type of database called SQLite)
- How we'll open and close the drawers (connecting to and disconnecting from the database)
- How we'll arrange the folders inside (setting up the basic structure)
This file is essential because it creates the foundation for storing all the information our library system will need.
- models.py
If database.py is the blueprint for our filing cabinet, models.py is like designing the different types of folders we'll put inside. In our library system, we need folders for:
- Books
- Authors
- Users
- Booklists
Each of these folders (we call them "models" in programming) has specific information we want to keep track of. For example:
- A Book folder might have slots for "title" and "author"
- An Author folder might have a slot for "name"
This file also defines how these folders relate to each other. For instance, it specifies that a Book can have multiple Authors, and an Author can have written multiple Books.
- schemas.py
While models.py defines how we store information, schemas.py defines how we present and accept information when communicating with users of our system.
Think of schemas as forms:
- When someone wants to add a new book, what information do they need to provide? That's an "input schema".
- When someone asks for information about a book, what details do we show them? That's an "output schema".
These schemas ensure that we're always providing and receiving information in a consistent, expected format, like a standardized form.
- main.py
This is where all the action happens! If our library system was a restaurant:
- database.py would be the kitchen setup
- models.py would be the recipe book
- schemas.py would be the menu
- main.py would be the waitstaff
main.py defines all the actions our library system can perform, like:
- Adding a new book
- Listing all books
- Finding a specific book
- Creating a new user
- Making a booklist
Each of these actions is called an "endpoint". When someone wants to interact with our library system (like adding a book or searching for an author), main.py handles their request, goes to the database if necessary, and returns the appropriate response.
In essence, main.py brings together all the other components to create a functioning library management system. It's the file that makes everything work together smoothly.
Step 3: Set up the database
database.py:
from sqlalchemy import create_engine
from sqlalchemy.ext.declarative import declarative_base
from sqlalchemy.orm import sessionmaker
SQLALCHEMY_DATABASE_URL = "sqlite:///./library.db"
engine = create_engine(SQLALCHEMY_DATABASE_URL, connect_args={"check_same_thread": False})
SessionLocal = sessionmaker(autocommit=False, autoflush=False, bind=engine)
Base = declarative_base()
def get_db():
db = SessionLocal()
try:
yield db
finally:
db.close()
Let's dive into database.py and explore how it might look with different database options, including PostgreSQL.
Explaining database.py:
Think of database.py as the blueprint for your data storage system. It's like deciding what kind of shelving system you'll use in your library before you start putting books on the shelves.
Key components in database.py:
- Database URL: This is like the address of your storage facility.
- Engine: This is the machinery that connects your application to the database.
- SessionLocal: This is like a temporary workspace where you can organize books before putting them on shelves.
- Base: This is a template for creating your data models (like defining what information you'll store about each book).
Now, let's look at how this might change with different databases:
- SQLite (as in the original example):
SQLite is like a small, personal bookshelf. It's great for small projects or testing.
SQLALCHEMY_DATABASE_URL = "sqlite:///./library.db"
engine = create_engine(SQLALCHEMY_DATABASE_URL, connect_args={"check_same_thread": False})
- PostgreSQL:
PostgreSQL is like a large, industrial-strength shelving system. It's great for bigger projects and can handle many people accessing it at once.
SQLALCHEMY_DATABASE_URL = "postgresql://user:password@localhost/dbname"
engine = create_engine(SQLALCHEMY_DATABASE_URL)
- MySQL:
MySQL is another popular option, similar to PostgreSQL in scale.
SQLALCHEMY_DATABASE_URL = "mysql://user:password@localhost/dbname"
engine = create_engine(SQLALCHEMY_DATABASE_URL)
The main differences are:
- The database URL: This changes based on the type of database, where it's located, and how to access it.
- Engine creation: Some databases might need extra arguments when creating the engine.
Here's a full example of database.py using PostgreSQL:
from sqlalchemy import create_engine
from sqlalchemy.ext.declarative import declarative_base
from sqlalchemy.orm import sessionmaker
# PostgreSQL database URL
SQLALCHEMY_DATABASE_URL = "postgresql://user:password@localhost/library_db"
# Create the SQLAlchemy engine
engine = create_engine(SQLALCHEMY_DATABASE_URL)
# Create a SessionLocal class
SessionLocal = sessionmaker(autocommit=False, autoflush=False, bind=engine)
# Create a Base class
Base = declarative_base()
# Dependency to get the database session
def get_db():
db = SessionLocal()
try:
yield db
finally:
db.close()
The main changes for PostgreSQL are:
- The database URL now points to a PostgreSQL database.
- We don't need the `connect_args={"check_same_thread": False}` that was specific to SQLite.
Everything else remains largely the same. This is one of the benefits of using an ORM (Object-Relational Mapping) like SQLAlchemy - much of your code can remain the same even if you switch databases.
Remember, if you're using PostgreSQL, you'll need to:
- Have PostgreSQL installed on your system or have access to a PostgreSQL server.
- Create the database before running your application.
- Install the appropriate Python database driver (in this case, `psycopg2`).
You would install the necessary packages with:
`pip install sqlalchemy psycopg2`
This setup allows your application to connect to and interact with a PostgreSQL database, providing a robust solution for storing and retrieving your library data.
A quick introduction to ORMs:
ORM (Object-Relational Mapping)
Imagine you're trying to communicate with someone who speaks a different language. An ORM is like a translator that helps your Python code "talk" to your database, which speaks its own language (SQL).
Key points about ORMs:
- Bridge between objects and tables: ORMs allow you to work with database tables as if they were Python objects.
- Language abstraction: You can write Python code instead of SQL queries.
- Database independence: You can switch databases without changing much of your code.
- Simplifies data operations: Makes it easier to create, read, update, and delete data.
SQLAlchemy
SQLAlchemy is one of the most popular ORMs for Python. It's like a Swiss Army knife for database operations.
Key features of SQLAlchemy:
- Powerful ORM capabilities: Translates Python classes to database tables and vice versa.
- SQL Expression Language: Allows you to write SQL-like expressions using Python constructs.
- Database agnostic: Works with many databases (PostgreSQL, MySQL, SQLite, etc.) with minimal code changes.
- Connection pooling: Efficiently manages database connections.
- Transaction management: Helps ensure data integrity during operations.
How SQLAlchemy Works:
Defining Models: You define Python classes that represent your database tables. For example:
from sqlalchemy import Column, Integer, String
from sqlalchemy.ext.declarative import declarative_base
Base = declarative_base()
class Book(Base):
__tablename__ = 'books'
id = Column(Integer, primary_key=True)
title = Column(String)
author = Column(String)
This class represents a 'books' table with id, title, and author columns.
Creating Tables: SQLAlchemy can create the actual database tables based on your model definitions:
Base.metadata.create_all(engine)
Performing Operations: Instead of writing SQL, you use Python methods:
# Creating a new book
new_book = Book(title="1984", author="George Orwell")
session.add(new_book)
session.commit()
# Querying books
books = session.query(Book).filter(Book.author == "George Orwell").all()
Relationships: SQLAlchemy makes it easy to define relationships between tables:
class Author(Base):
__tablename__ = 'authors'
id = Column(Integer, primary_key=True)
name = Column(String)
books = relationship("Book", back_populates="author")
class Book(Base):
__tablename__ = 'books'
id = Column(Integer, primary_key=True)
title = Column(String)
author_id = Column(Integer, ForeignKey('authors.id'))
author = relationship("Author", back_populates="books")
Benefits of Using SQLAlchemy:
- Productivity: Write less code to achieve database operations.
- Maintainability: Changes to the database schema can often be made by just updating the Python models.
- Security: Helps prevent SQL injection attacks by properly escaping parameters.
- Flexibility: Easy to switch between different databases.
- Learning Curve: While powerful, it has a steeper learning curve compared to simpler ORMs.
- Connection Pooling: SQLAlchemy efficiently manages database connections, reusing them to improve performance.
- Query Optimization: SQLAlchemy can optimize queries, sometimes generating more efficient SQL than a developer might write manually.
In the context of our Library Management System, SQLAlchemy allows us to define our books, authors, users, and booklists as Python classes, and then interact with them using Python code, without having to write raw SQL queries. This makes the code more readable, maintainable, and portable across different database systems.
Step 4: Define models
In models.py:
from sqlalchemy import Column, Integer, String, ForeignKey, Table
from sqlalchemy.orm import relationship
from database import Base
book_author = Table('book_author', Base.metadata,
Column('book_id', Integer, ForeignKey('books.id')),
Column('author_id', Integer, ForeignKey('authors.id'))
)
class Book(Base):
__tablename__ = "books"
id = Column(Integer, primary_key=True, index=True)
title = Column(String, index=True)
authors = relationship("Author", secondary=book_author, back_populates="books")
class Author(Base):
__tablename__ = "authors"
id = Column(Integer, primary_key=True, index=True)
name = Column(String, index=True)
books = relationship("Book", secondary=book_author, back_populates="authors")
class User(Base):
__tablename__ = "users"
id = Column(Integer, primary_key=True, index=True)
username = Column(String, unique=True, index=True)
email = Column(String, unique=True, index=True)
class Booklist(Base):
__tablename__ = "booklists"
id = Column(Integer, primary_key=True, index=True)
name = Column(String, index=True)
user_id = Column(Integer, ForeignKey('users.id'))
user = relationship("User", back_populates="booklists")
books = relationship("Book", secondary="booklist_book")
User.booklists = relationship("Booklist", back_populates="user")
booklist_book = Table('booklist_book', Base.metadata,
Column('booklist_id', Integer, ForeignKey('booklists.id')),
Column('book_id', Integer, ForeignKey('books.id'))
)
Step 5: Define Pydantic schemas
In schemas.py:
from pydantic import BaseModel
from typing import List, Optional
class AuthorBase(BaseModel):
name: str
class AuthorCreate(AuthorBase):
pass
class Author(AuthorBase):
id: int
class Config:
orm_mode = True
class BookBase(BaseModel):
title: str
class BookCreate(BookBase):
author_ids: List[int]
class Book(BookBase):
id: int
authors: List[Author]
class Config:
orm_mode = True
class UserBase(BaseModel):
username: str
email: str
class UserCreate(UserBase):
password: str
class User(UserBase):
id: int
class Config:
orm_mode = True
class BooklistBase(BaseModel):
name: str
class BooklistCreate(BooklistBase):
user_id: int
class Booklist(BooklistBase):
id: int
user: User
books: List[Book]
class Config:
orm_mode = True
Step 6: Implement API endpoints
In main.py:
from fastapi import FastAPI, Depends, HTTPException
from sqlalchemy.orm import Session
import models, schemas, database
models.Base.metadata.create_all(bind=database.engine)
app = FastAPI()
# Book endpoints
@app.post("/api/books/", response_model=schemas.Book)
def create_book(book: schemas.BookCreate, db: Session = Depends(database.get_db)):
db_book = models.Book(title=book.title)
for author_id in book.author_ids:
author = db.query(models.Author).filter(models.Author.id == author_id).first()
if author:
db_book.authors.append(author)
db.add(db_book)
db.commit()
db.refresh(db_book)
return db_book
@app.get("/api/books/", response_model=List[schemas.Book])
def read_books(skip: int = 0, limit: int = 100, db: Session = Depends(database.get_db)):
books = db.query(models.Book).offset(skip).limit(limit).all()
return books
@app.get("/api/books/{book_id}", response_model=schemas.Book)
def read_book(book_id: int, db: Session = Depends(database.get_db)):
book = db.query(models.Book).filter(models.Book.id == book_id).first()
if book is None:
raise HTTPException(status_code=404, detail="Book not found")
return book
# Author endpoints
@app.post("/api/authors/", response_model=schemas.Author)
def create_author(author: schemas.AuthorCreate, db: Session = Depends(database.get_db)):
db_author = models.Author(name=author.name)
db.add(db_author)
db.commit()
db.refresh(db_author)
return db_author
@app.get("/api/authors/", response_model=List[schemas.Author])
def read_authors(skip: int = 0, limit: int = 100, db: Session = Depends(database.get_db)):
authors = db.query(models.Author).offset(skip).limit(limit).all()
return authors
# User endpoints
@app.post("/api/users/", response_model=schemas.User)
def create_user(user: schemas.UserCreate, db: Session = Depends(database.get_db)):
db_user = models.User(username=user.username, email=user.email)
db.add(db_user)
db.commit()
db.refresh(db_user)
return db_user
@app.get("/api/users/", response_model=List[schemas.User])
def read_users(skip: int = 0, limit: int = 100, db: Session = Depends(database.get_db)):
users = db.query(models.User).offset(skip).limit(limit).all()
return users
# Booklist endpoints
@app.post("/api/booklists/", response_model=schemas.Booklist)
def create_booklist(booklist: schemas.BooklistCreate, db: Session = Depends(database.get_db)):
db_booklist = models.Booklist(**booklist.dict())
db.add(db_booklist)
db.commit()
db.refresh(db_booklist)
return db_booklist
@app.get("/api/booklists/", response_model=List[schemas.Booklist])
def read_booklists(skip: int = 0, limit: int = 100, db: Session = Depends(database.get_db)):
booklists = db.query(models.Booklist).offset(skip).limit(limit).all()
return booklists
# Search endpoint
@app.get("/api/search/books")
def search_books_by_author(author_name: str, db: Session = Depends(database.get_db)):
books = db.query(models.Book).join(models.Book.authors).filter(models.Author.name.ilike(f"%{author_name}%")).all()
return books
Step 7: Run the API
Run the following command in your terminal:
`uvicorn main:app --reload`
Your API should now be running at http://127.0.0.1:8000
Testing the API:
- Using Postman:
- Open Postman
- Set the HTTP method (GET, POST, etc.) and enter the URL (e.g., http://127.0.0.1:8000/api/books/)
- For POST requests, go to the "Body" tab, select "raw" and "JSON", and enter the request body
- Click "Send" to make the request
Test using Postman
Example POST request to create a book: URL: http://127.0.0.1:8000/api/books/ Method: POST
Body:
{
"title": "1984",
"author_ids": [1]
}
- Using curl:
GET request:
`curl http://127.0.0.1:8000/api/books/`
POST request:
`curl -X POST -H "Content-Type: application/json" -d '{"title": "1984", "author_ids": [1]}' http://127.0.0.1:8000/api/books/`
Search request:
`curl "http://127.0.0.1:8000/api/search/books?author_name=Orwell"`
This implementation covers the basic CRUD operations for books, authors, users, and booklists, as well as a simple search functionality.